The gallery with photo-filters
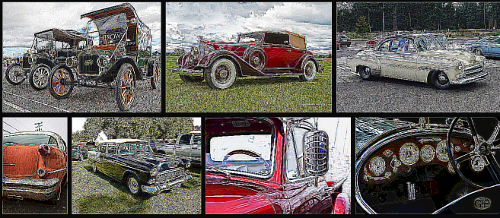
Today I want to continue talking about Moon Gallery jQuery library. It’s time to consider photo-filters.
There are two ways to transform images in JavaScript:
1. You can draw a canvas element and replace your image with it.
2. Or you can use SVG-filters.
First way consumes significantly more resources, but it’s supported by almost all modern browsers.
Second way is preferable, but it’s not supported by old Android devices.
Moon Gallery supports both technics. You can even set your gallery so that SVG-filters will be used if a browser supports them, otherwise canvas will be used.
Canvas filters
The library supports these canvas filters:
-
grayscale
canvasFilters: [['grayscale']]
-
brightness
canvasFilters: [['brightness', [20]]]
-
sepia
canvasFilters: [['sepia']]
-
contrast
canvasFilters: [['contrast', [20]]]
-
vibrance
canvasFilters: [['vibrance', [20]]]
-
saturate
canvasFilters: [['saturate', [20]]]
-
colorize
canvasFilters: [['colorize', [25, 150, 50, 25]]]
-
noise
canvasFilters: [['noise', [25]]]
- You can use
Combination of canvas filters
canvasFilters: [ ['colorize', [25, 150, 50, 25]], ['brightness', [-15]], ['contrast', [-15]], ['vibrance', [50]] ]
SVG filters
If we say about canvas there is the limited list of filters which you can use. There is no such list of SVG-filters. To use some SVG-filter you should add an SVG-element with a filter to your page, and then specify the id in the settings.
-
-
-
grayscale
MMG.Gallery('Simple', { url: 'assets/simple.json', svgFiltersId: 'grayscale' } );
You shouldn’t add any SVG element in the markup because it generated automatically in this case.
-
sepia
in the script:
MMG.Gallery('Simple', { url: 'assets/simple.json', svgFiltersId: 'sepia' }
in markup:
<svg width="0" height="0"> <defs> <filter id="sepia"> <fecolormatrix type="matrix" values="0.343 0.669 0.119 0 0 0.249 0.626 0.130 0 0 0.172 0.334 0.111 0 0 0 0 0 1 0"> </fecolormatrix> </filter> </defs> </svg>
-
saturate
in the script:
MMG.Gallery('Simple', { url: 'assets/simple.json', svgFiltersId: 'saturate' }
in markup:
<svg width="0" height="0"> <defs> <filter id="saturate"> <fecolormatrix type="saturate" values="0.4"> </filter> </defs> </svg>
-
contrast
in the script:
MMG.Gallery('Simple', { url: 'assets/simple.json', svgFiltersId: 'contrast' }
in markup:
<svg width="0" height="0"> <defs> <filter id="contrast"> <fecomponenttransfer> <fefuncr type="linear" slope="1.5" intercept="-0.25"></fefuncr> <fefuncg type="linear" slope="1.5" intercept="-0.25"></fefuncg> <fefuncb type="linear" slope="1.5" intercept="-0.25"></fefuncb> </fecomponenttransfer> </filter> </defs> </svg>
-
brightness
in the script:
MMG.Gallery('Simple', { url: 'assets/simple.json', svgFiltersId: 'brightness' }
in markup:
<svg width="0" height="0"> <defs> <filter id="brightness"> <fecomponenttransfer> <fefuncr type="linear" slope="2"></fefuncr> <fefuncg type="linear" slope="2"></fefuncg> <fefuncb type="linear" slope="2"></fefuncb> </fecomponenttransfer> </filter> </defs> </svg>
-
blur
in the script:MMG.Gallery('Simple', { url: 'assets/simple.json', svgFiltersId: 'blur' }
in markup:
<svg width="0" height="0"> <defs> <filter id="blur"> <fegaussianblur stddeviation="2"></fegaussianblur> </filter> </defs> </svg>
-
colorize
in the script:MMG.Gallery('Simple', { url: 'assets/simple.json', svgFiltersId: 'colorize' }
in markup:
<svg width="0" height="0"> <defs> <filter id="colorize"> <fecomponenttransfer> <fefuncr type="linear" slope="0"></fefuncr> <fefuncg type="linear" slope="1"></fefuncg> </fecomponenttransfer> </filter> </defs> </svg>
-
descrete
in the script:MMG.Gallery('Simple', { url: 'assets/simple.json', svgFiltersId: 'discrete' }
in markup:
<svg width="0" height="0"> <defs> <filter id="discrete"> <fecomponenttransfer> <fefuncr type="discrete" tablevalues="0 0.3 1"></fefuncr> <fefuncg type="discrete" tablevalues="0 0.8 1"></fefuncg> <fefuncb type="discrete" tablevalues="0 0.5 1"></fefuncb> </fecomponenttransfer> </filter> </defs> </svg>
-
invert
in the script:MMG.Gallery('Simple', { url: 'assets/simple.json', svgFiltersId: 'invert' }
in markup:
<svg width="0" height="0"> <defs> <filter id="invert"> <fecomponenttransfer> <fefuncr type="table" tablevalues="1 0"></fefuncr> <fefuncg type="table" tablevalues="1 0"></fefuncg> <fefuncb type="table" tablevalues="1 0"></fefuncb> </fecomponenttransfer> </filter> </defs> </svg>
-
noise
in the script:MMG.Gallery('Simple', { url: 'assets/simple.json', svgFiltersId: ''noise-1' }
in markup:
<svg width="0" height="0"> <defs> <filter id="noise-1"> <feconvolvematrix order="3" kernelmatrix="-1 -1 -1 -1 7 -1 -1 -1 -1"> </feconvolvematrix> </filter> </defs> </svg>
-
another noise
in the script:MMG.Gallery('Simple', { url: 'assets/simple.json', svgFiltersId: 'noise' }
in markup:
<svg width="0" height="0"> <defs> <filter id="noise"> <feconvolvematrix order="3" kernelmatrix="1 -1 1 -1 -0.1 -1 1 -1 1"> </feconvolvematrix> </filter> </defs> </svg>
-
combination
in the script:MMG.Gallery('Simple', { url: 'assets/simple.json', svgFiltersId: 'combo-1' }
in markup:
<svg width="0" height="0"> <defs> <filter id="combo-1"> <fegaussianblur stddeviation="1" result="a1"> <fecolormatrix in="a1" type="saturate" values="0"> </filter> </defs> </svg>
-
-
As you can see, SVG gives more opportunities than canvas. Moreover, SVG-filters are faster.
Page with Comments